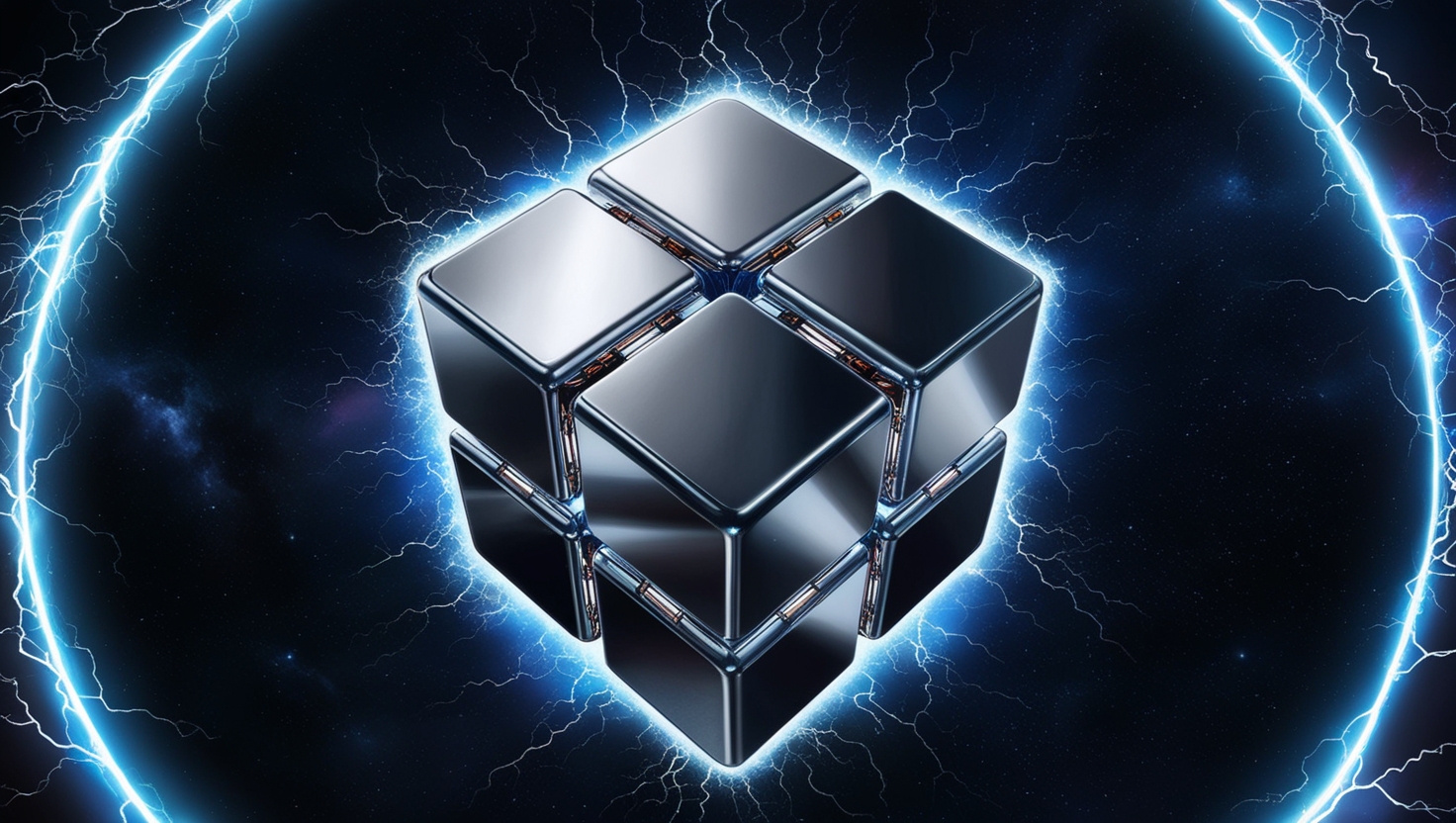
Understanding the 4D Hypercube: A Journey into the Fourth Dimension
Have you ever wondered what it would be like to see in four dimensions? While our brains are wired to understand three-dimensional space, mathematicians and computer scientists have developed ways to visualize higher dimensions. In this article, we’ll explore the fascinating world of the 4D hypercube (tesseract) and break down how we can visualize it on a 2D screen.
What is the Fourth Dimension?
Before diving into the hypercube, let’s understand what we mean by the fourth dimension. Just as a cube has width (x), height (y), and depth (z), a hypercube adds a fourth spatial dimension we’ll call ‘w’. While we can’t directly experience this dimension, we can understand it mathematically and project it into our familiar 3D space.
Think of it this way:
- A point is 0D (no dimensions)
- A line is 1D (length)
- A square is 2D (length and width)
- A cube is 3D (length, width, and height)
- A hypercube is 4D (length, width, height, and an additional perpendicular direction)
The Structure of a Hypercube
A hypercube consists of:
- 16 vertices (corner points)
- 32 edges (lines connecting vertices)
- 24 faces (square surfaces)
- 8 cubic cells (3D cubes)
In our visualization, we represent this structure using:
- Colored vertices as points
- Gradient-colored edges connecting related vertices
- A convex hull showing the outer shape of the projection
The Mathematics of 4D Rotation
The most fascinating aspect of our visualization is the rotation in 4D space. Here’s how it works:

1. 4D Coordinates
Each vertex of the hypercube is represented by four coordinates (x, y, z, w). In our code, we generate these coordinates using binary patterns:
for (let i = 0; i < 16; i++) {
const x = (i & 1) ? 1 : -1;
const y = (i & 2) ? 1 : -1;
const z = (i & 4) ? 1 : -1;
const w = (i & 8) ? 1 : -1;
vertices.push([x, y, z, w]);
}
2. Rotation Matrices
We rotate the hypercube in multiple planes simultaneously:
- XW plane (analogous to rotating in a plane perpendicular to our 3D space)
- YW plane (another 4D rotation)
- YZ plane (a familiar 3D rotation)
The rotation is performed using these mathematical transformations:
const cosA = Math.cos(angle * rotationModifier.x);
const sinA = Math.sin(angle * rotationModifier.w);
let x1 = x * cosA - w * sinA;
let w1 = x * sinA + w * cosA;
3. Projection Pipeline
To display the 4D object on our 2D screen, we use a series of projections:
- 4D to 3D Projection: We project the 4D points onto a 3D hyperplane using the method:
const dot = x1 + y1 + z1 + w1;
let x2 = x1 - dot/4;
let y2 = y1 - dot/4;
let z2 = z1 - dot/4;
let w2 = w1 - dot/4;
- 3D to 2D Projection: We then project the resulting 3D points onto our 2D screen:
const X = (x2 - y2) / Math.SQRT2;
const Y = (z2 - w2) / Math.SQRT2;
Interactive Features
Our visualization includes several interactive features:
- Mouse Control: Moving your mouse affects the rotation speed and direction:
- Horizontal movement influences X and Z rotations
- Vertical movement influences Y and W rotations
- Visibility Toggles: Users can show/hide:
- Coordinate labels
- Vertices (nodes)
- Edges (connecting lines)
- Animation Control: Play/pause functionality to freeze the rotation at any point
Technical Implementation
The visualization is built using modern web technologies:
- SVG for rendering the geometry
- JavaScript for calculations and animations
- Tailwind CSS for the user interface
- Linear gradients for edge visualization
- CSS 3D transforms for the flip card effect
Understanding Through Analogy
To better understand 4D space, consider this analogy: Imagine a 2D being (like a flat paper character) trying to understand our 3D world. They can only see 2D slices of 3D objects. Similarly, what we see in our visualization are 3D “slices” of the 4D hypercube as it rotates through the fourth dimension.
Conclusion
While we can’t directly perceive four spatial dimensions, mathematical visualizations like this hypercube help us understand higher-dimensional geometry. The combination of mathematical principles, computer graphics, and interactive controls creates a window into a world beyond our natural perception.
The next time you interact with the visualization, remember that you’re not just looking at an abstract animation – you’re seeing a glimpse of how mathematicians and scientists understand and work with higher dimensions in fields ranging from theoretical physics to data science.