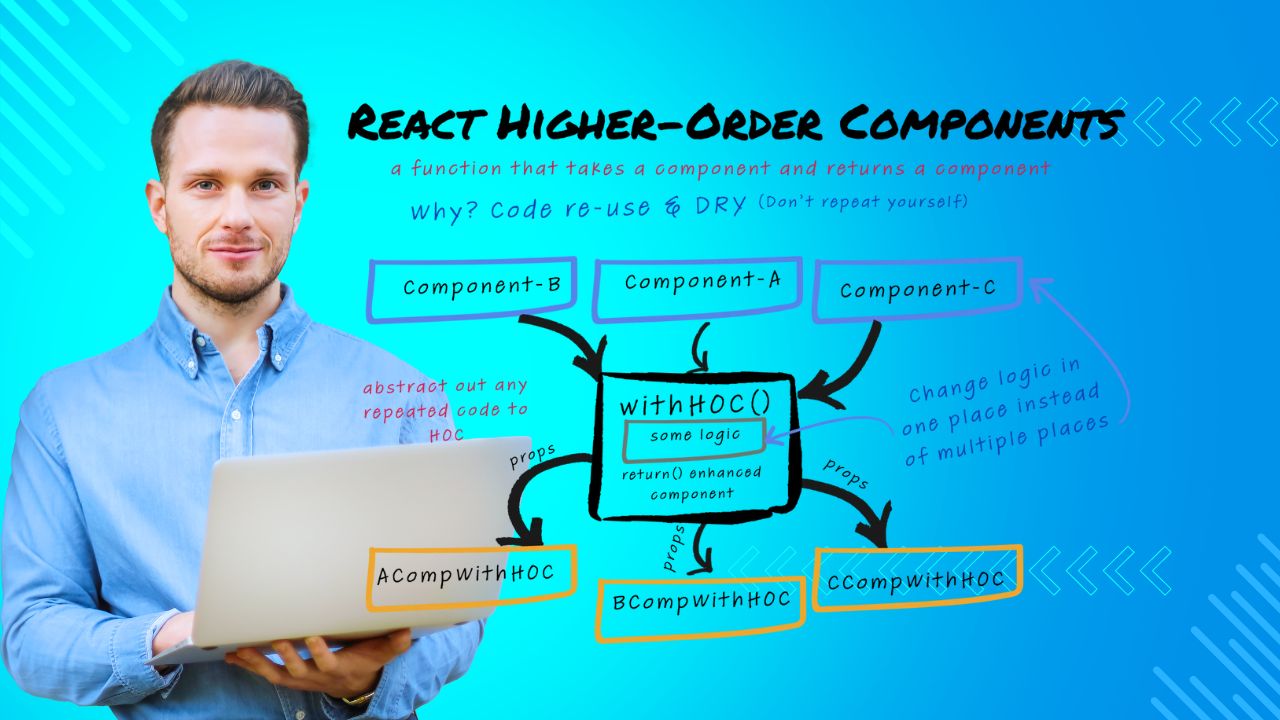
Higher Order Components in ReactJS : How to setup a simple NextJS SSR Authentication
The Context
ReactJS offers a compelling pattern with Higher Order Components (HOCs), which allows developers to enhance their components with added features. When utilized in NextJS applications, HOCs prove invaluable for creating effective authentication systems. They enable the construction of sophisticated authentication frameworks that both secure routes and efficiently manage user access. This method provides a seamless integration of access control into user experiences without sacrificing component modularity or the scalability of the application’s architecture.
Demystifying Higher Order Components in React
Within the React ecosystem, Higher Order Components (HOCs) stand as a pivotal concept for enhancing code modularity and reusability. These advanced constructs allow developers to encapsulate shared behavior across multiple components through wrapping functions. An HOC receives a component and returns a new one endowed with additional capabilities, thereby enabling the widespread application of logic without redundant code.
Higher Order Components operate analogously to decorators, extending function functionality without altering their inherent structure. By utilising an HOC, React developers can infuse extra props and modify the behavior of the underlying component, thus preserving the component’s original contract while bestowing new attributes or states. A prevalent application for HOCs is in conducting authentication checks. For example, they can efficiently enforce authentication logic consistently across various components or pages of a React app—making them an ideal choice for such tasks.
In the context of Next.js, which builds upon React, HOCs can significantly simplify the application of authentication across pages. Imagine designing a singular HOC that handles authentication checks. This HOC can be readily applied to disparate page components by wrapping them, thereby establishing access control without redundant authentication logic. In effect, as users move through an application’s pages, the HOC preliminarily processes their authentication status, granting or restricting component access accordingly. This abstraction of auth state management results in a cleaner, more maintainable codebase.
This code below for example defines a higher-order component (HOC) in a React and Next.js application that adds an authentication layer to other components. It works by first importing necessary utilities for handling cookies, JWT verification, and redirection. The Auth
function wraps around a component (WrappedComponent
) to enhance it with authentication logic.
When the new component (Authenticated
) is rendered, it attempts to fetch a JWT (JSON Web Token) cookie named ‘jwt’ using getCookie
. The fetched cookie is then passed to verifyJWT
, a function imported from a local module, which checks if the JWT is valid. If verifyJWT
returns undefined
, indicating that the JWT is either invalid or not present, the function then triggers a redirection to the login page using redirect
from next/navigation
. This ensures the user is authenticated before accessing the component. If the JWT is valid, the original WrappedComponent
is rendered with all its passed props, allowing the authenticated user access. This setup is particularly useful for protecting routes and components that require user authentication.
import React from 'react';
import { getCookie } from 'cookies-next';
import { cookies } from 'next/headers';
import { redirect } from 'next/navigation';
import {verifyJWT } from '../auth/jwt/'
const Auth = (WrappedComponent: React.FC<any>) => {
return function Authenticated(props: any) {
// Assuming the server-side or statically prepared cookie context
const cookie = verifyJWT(getCookie('jwt', { cookies }));
if (cookie === undefined) {
// Using next/navigation for a server-compatible redirect
return redirect('/auth/signin');
}
// Render the wrapped component if the cookie is present
return <WrappedComponent {...props} />;
};
};
export default Auth;
This is how you implement it:
import MainPage from "@/components/Dashboard/MainPAge";
import { Metadata } from "next";
import DefaultLayout from "@/components/Layouts/DefaultLayout";
import Auth from "./auth/auth";
export const metadata: Metadata = {
title:
"Next.js My Dashboard | Domenico Rutigliano Digital Transformation Consultant - Next.js Dashboard Template",
description: "This is Next.js Home for Domenico Rutigliano Dashboard Template",
};
const Home = () => {
return (
<DefaultLayout>
<MainPage />
</DefaultLayout>
);
}
export default Auth(Home);
With this approach, you can select which pages are behind the login wall and which ones are public by either hardcoding it or applying some logic to dynamically choose in the backend.
Not restricted to authentication alone, HOCs play a vital role in standardising other reusable logic such as data fetching, analytics integration, and theme customisation. The agility they offer is instrumental in developing scalable and maintainable applications. It is, however, crucial to comprehend their architecture to sidestep issues like prop collisions and unwarranted component hierarchy re-rendering. Nevertheless, a judiciously implemented HOC augments the React development process, unlocking extensive potential for logic reusability in components.
To conclude, Higher Order Components are an integral aspect of React’s design philosophy, offering an advanced yet approachable means to create reusable components. They promote a clean and DRY (Don’t Repeat Yourself) methodology for disseminating functionality across various application segments. By combining the compositional power of React with the abstracting capability of HOCs, developers can masterfully integrate complex logic with user-friendly simplicity. Mastery of HOCs is synonymous with enhanced ability in crafting robust, scalable, and effective React applications.
Building a Secure NextJS Application: Implementing Authenticated Routes with HOCs
Establishing a robust and secure front end with proper authentication demands a meticulous and systematic strategy, especially when utilising NextJS, a favoured framework within the React ecosystem. A Higher Order Component (HOC), a pattern in React that promotes the reuse of component logic, emerges as a fundamental tool for protecting routes. This tutorial delineates the process of crafting an HOC to enforce authentication on NextJS pages.
Understanding an HOC is pivotal—it is a function that accepts a component and returns a new component, essentially ‘wrapping’ the original. This wrapper is capable of injecting props or enforcing logic like redirecting unauthenticated users. When applied to NextJS, we create a security layer that enshrouds our pages, acting as a vigilant gatekeeper against unauthorised access.
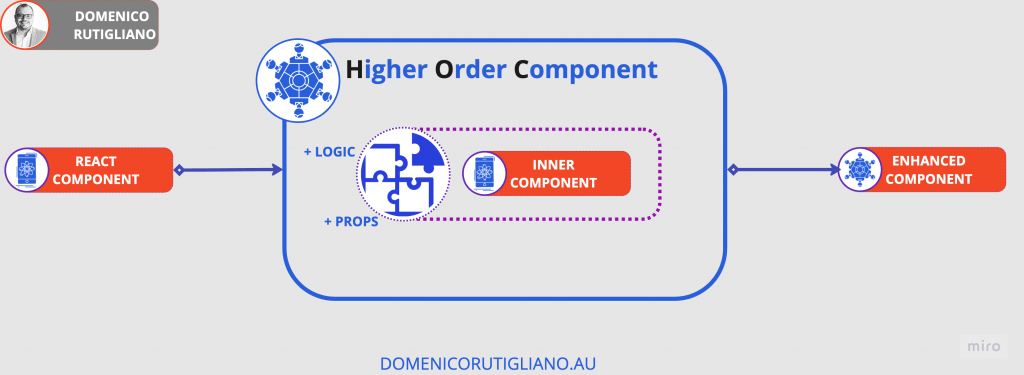
To create our authentication-focused HOC, we commence by formulating a function that receives a React component, along with additional parameters like role permissions. This function then returns a new component that envelops the original. In TypeScript, we enhance our HOC to ensure type safety by rigorously annotating component and parameter types, thus fortifying our code and minimizing runtime errors.
The bedrock of our HOC exploits NextJS’s ‘getInitialProps’ or ‘getServerSideProps’ lifecycle methods to confirm authentication status pre-render. These methods spring into action server-side for initial page loads or client-side during navigation, allowing us to consult our authentication service for the user’s login status; if the verdict is negative, we redirect them to an alternate course.
Integral to our HOC’s design is adept error handling. Should the user verification fail or denote unauthentication, our HOC is primed to either reroute users to a login screen or present a suitable error message—this must be executed smoothly and without disrupting user experience.
In summation, securing NextJS pages with an HOC involves a meticulously defined wrapper function equipped with TypeScript annotations, strategic implementation of NextJS lifecycle methods for pre-render checks, elegant error handling, and the redirection of unauthorized access. This results in a stalwart shield encasing our sensitive components, upholding information security and winning user confidence.
Conclusively, developing a NextJS HOC for page authentication is manageable with a clear TypeScript foundation, strategic parameter management, and skillful error resolution, all of which contribute to a secure and uninterrupted user experience.
Applying Wisdom: Leveraging HOCs in NextJS
Higher-Order Components (HOCs) are design patterns in React and its frameworks like NextJS that enhance components with new capabilities, without changing their original logic. They shine in tasks such as user authentication and user experience enhancements. In NextJS, HOCs can wrap logical structures around components to enforce login restrictions. An authentication HOC, for instance, enables developers to control access to any component by wrapping it with this HOC. It conducts checks before rendering the wrapped component, confirming that users meet specific criteria, such as having authentication.
In its implementation, an HOC typically intercepts the rendering process using NextJS’s lifecycle methods or hooks, like ‘getInitialProps’ or ‘getServerSideProps’. Whether during server-side rendering or client-side hydration, these methods assess user permissions to access a page. If the user fails to meet the conditions, the HOC redirects them to a sign-in page or indicates restricted access. This not only improves user experience by preventing unauthorised access but also promotes code reusability and adherence to the “Don’t Repeat Yourself” (DRY) principle.
Consider an HOC named ‘withAuth’ applied to a user profile page component. It evaluates the user’s authentication status before allowing the page to render normally. If unauthenticated, it redirects to a login page. This maintains a separation of concerns by keeping the profile component free from authentication logic. Moreover, the HOC can provide authentication-related props to the component, supplying user information when needed.
Smart use of HOCs in NextJS leads to cleaner, maintainable, and secure code. As the web becomes more personal and privacy concerns mount, HOCs become essential in a developer’s toolkit, balancing functionality with user-focused design principles. Applying HOCs in NextJS exemplifies the union of developer efficiency with an enhanced user experience, showcasing the practical wisdom of advanced web development practices.
Conclusion
In summary, the adoption of Higher Order Components (HOCs) in NextJS demonstrates their significant efficiency and elegance, particularly in the realm of authentication management. These HOCs confer upon developers a powerful means to embed authentication processes within multiple components, enhancing user security while preserving the seamless operation of the application’s framework. Implementing HOCs within NextJS applications effectively capitalizes on the framework’s robust features for enforcing access control—proving to be strategically advantageous. Beyond the encapsulation of authentication logic, HOCs commendably advance code reusability and promote a more organized codebase. In reflecting upon the key points discussed in the article, the indispensable role of HOCs in the framework of advanced NextJS applications is reiterated, underscoring their essential contribution to crafting resilient, well-structured software.